



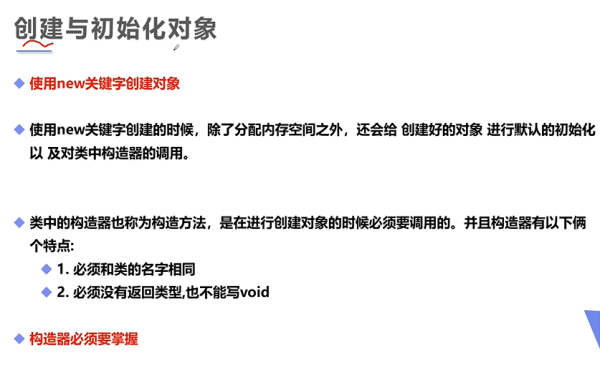
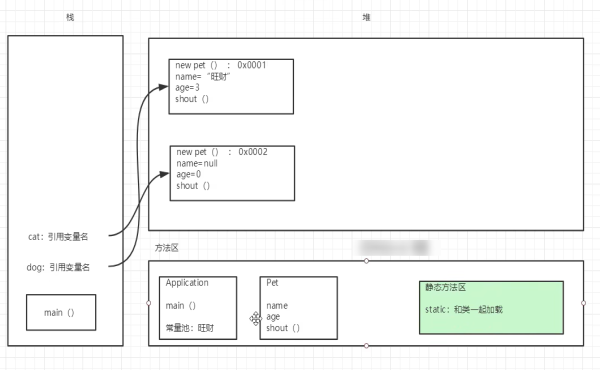
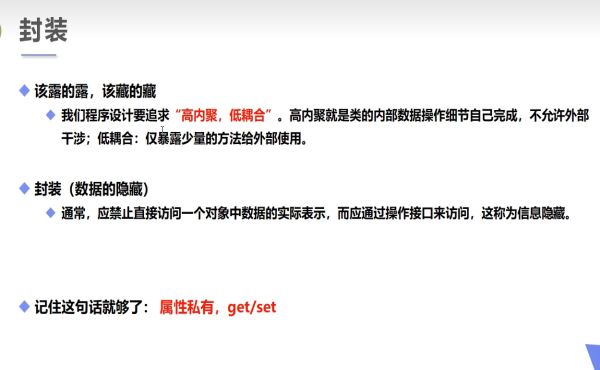
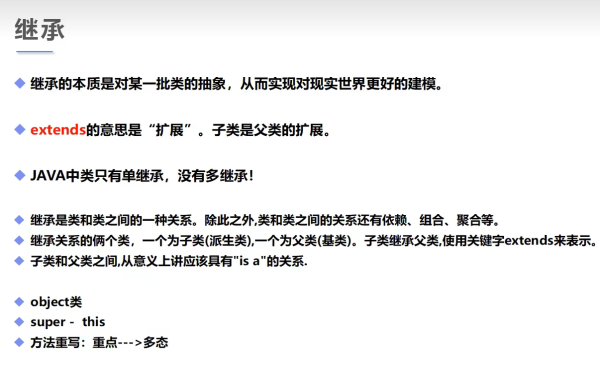

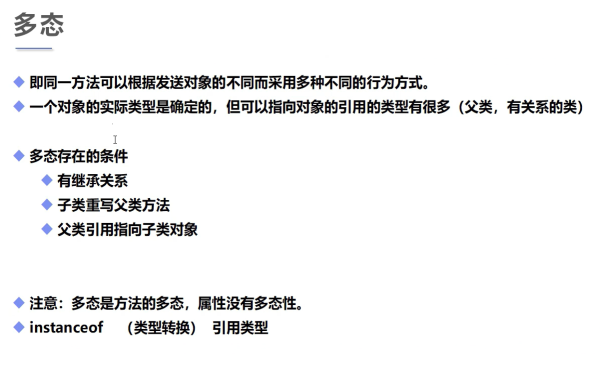
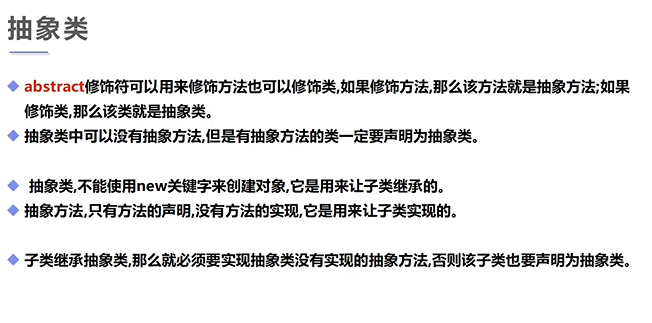
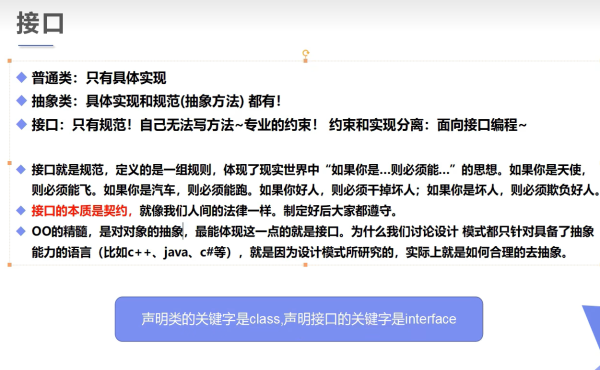
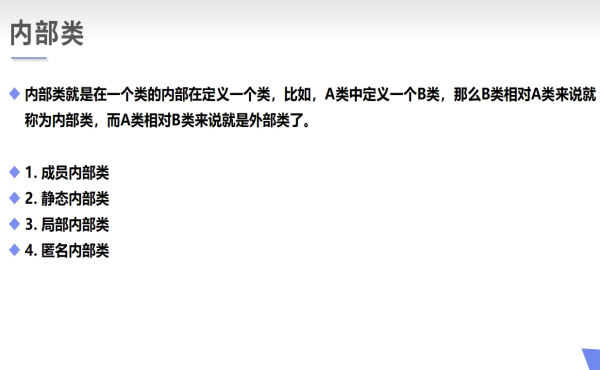
package top.oop.demo01;
import java.io.IOException;
//Demo01 类
public class Demo01 {
//main 方法
public static void main(String[] args) {
}
/*
修饰符 返回值类型 方法名(...){
//方法体
return 返回值;
}
*/
//return 结束方法,返回一个结果!
public String sayHello(){
return "hello,world";
}
public void print(){ //void 表示为空,返回为空
return;
}
public int max(int a,int b){
return a>b ? a:b;//三元运算符 如果a>b 那么就返回a,否则返回b
}
//数组下标越界: Arrayindexoutofbounds
public void readFile(String file) throws IOException{
}
}
package top.oop.demo01;
public class Demo02 {
//静态方法 static
// public static void main(String[] args) {
// Student.say();
// }
//非静态方法
public static void main(String[] args) {
//实例化这个类new
//对象类型 对象名 = 对象值
Student student = new Student();
student.say();
}
// 和类一起加载的
public static void a(){
}
// 类实例化 之后才存在
public void b(){
}
}
package top.oop.demo01;
public class Demo03 {
public static void main(String[] args) {
//如果没有static,请new一个方法 int add = new Demo03().add(1, 2);
//实际参数和形式参数的类型要对应!
int add = Demo03.add(1, 2);
System.out.println(add);
}
public static int add(int a,int b ){
return a+b;
}
}
package top.oop.demo01;
import top.iyunsir.base.Demo03;
//值传递
public class Demo04 {
public static void main(String[] args) {
int a = 1;
System.out.println(a);
Demo04.change(a);
System.out.println(a);//1
}
public static void change(int a){
a = 10;
}
}
package top.oop.demo01;
// 引用传递: 对象,本质还是值传递
// 对象, 内存!
public class Demo05 {
public static void main(String[] args) {
Person person = new Person();
System.out.println(person.name);//null
Demo05.change(person);
System.out.println(person.name); //秦疆
}
public static void change(Person person){
//person是一个对象: 指向的 --> Person person = new Person();这是一个具体的人,可以改变属性!
person.name = "秦疆";
}
}
//定义了一个person类, 有一个属性: name
class Person{
String name; //null
}
package top.oop.demo01;
// 引用传递: 对象,本质还是值传递
// 对象, 内存!
public class Demo05 {
public static void main(String[] args) {
Person person = new Person();
System.out.println(person.name);//null
Demo05.change(person);
System.out.println(person.name); //秦疆
}
public static void change(Person person){
//person是一个对象: 指向的 --> Person person = new Person();这是一个具体的人,可以改变属性!
person.name = "秦疆";
}
}
//定义了一个person类, 有一个属性: name
class Person{
String name; //null
}
package top.oop.demo01;
//学生类
public class Student {
//静态方法方法
// public static void say(){
// System.out.println("学生说话了");
// }
//非静态方法
public void say(){
System.out.println("学生说话了");
}
}
package top.oop.demo02;
public class Application {
public static void main(String[] args){
//类: 抽象的,实例化
//类实例化后会返回一个自己的对象!
//student对象就是一个Student类的具体实例!
// Student student = new Student();
// student.study();
Student xiaoming = new Student();
Student xh = new Student();
xiaoming.name = "小明";
xiaoming.age = 21;
System.out.println(xiaoming.name);
System.out.println(xiaoming.age);
xh.name = "小红";
xh.age = 20;
System.out.println(xh.name);
System.out.println(xh.age);
}
}
package top.oop.demo02;
public class Application2 {
public static void main(String[] args) {
//new 实例化一个对象
Person person = new Person("iyunsir",27);
System.out.println(person.name);
}
}
package top.oop.demo02;
// java --->class
public class Person {
//一个类什么都不写,它也会存在一个方法
//显示的定义构造器
String name;
int age;
//实例化初始值
//1.使用new关键字,本质是在调用构造器
//2.用来初始化值
//3.默认构造器
// public Person(){
// this.name = "iyunsir";
// }
//有参构造: 一旦定义了有参构造,无参就必须显示定义
//重载
// public Person(String name){
// this.name = name;
// }
//alt+insert
public Person() {
}
public Person(String name) {
this.name = name;
}
public Person(String name, int age) {
this.name = name;
this.age = age;
}
}
/*
构造器:
1. 和类名相同
2. 没有返回值
作用:
1. new本质在调用构造方法
2. 初始化对象的值
注意点:
定义有参构造之后,如果想使用无参构造,显示的定义一个无参的构造
//alt+insert
*/
package top.oop.demo02;
public class Student {
//属性: 字段
String name;
int age;
//方法
public void study(){
System.out.println(this.name+"在学习");
}
}
//学程序好? 对世界进行更好的建模! --- 宅!
package top.oop.demo03;
public class Application {
public static void main(String[] args) {
Pet dog = new Pet();
dog.name = "旺财";
dog.age = 3;
dog.shout();
System.out.println(dog.name);
System.out.println(dog.age);
}
}
package top.oop.demo03;
public class Application2 {
public static void main(String[] args) {
/*
1. 类与对象
类是一个模板: 抽象, 对象是一个具体的实例
2. 方法
定义, 调用!
3. 对应的引用
引用类型: 基本类型(8)
对象是通过引用来操作的: 栈--->堆
4. 属性: 字端Field 成员变量
默认初始化:
数字: 0 0.0
char: u0000
boolean: false
引用: null
修饰符 属性类型 属性名 = 属性值!
5. 对象的创建和使用
- 必须使用new 关键字创造对象,构造器 Person iyunsir = new Person();
- 对象的属性 iyunsir.name
- 对象的方法 iyunsir.sleep()
6. 类:
静态的属性 属性
动态的行为 方法
封装,继承,多态
*/
}
}
package top.oop.demo03;
public class Pet {
public String name;
public int age;
// 无参构造
public void shout(){
System.out.println("叫了一声");
}
}
package top.oop.demo04;
public class Application {
public static void main(String[] args) {
Student s1 = new Student();
s1.setName("iyunsir");
// 方法名, 参数列表
System.out.println(s1.getName());
s1.setAge(-1);//不合法
System.out.println(s1.getAge());
}
}
package top.oop.demo04;
public class Student {
//属性私有
private String name; //名字
private int id; //学号
private char sex; //性别
private int age ; //年龄
// 提供一些可以操作这个属性的方法!
// 提供一个public的get,set方法
//get 得到这个数据
public String getName() {
return name;
}
//set 给这个数组设置值
public void setName(String name) {
this.name = name;
}
//alt + insert
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public char getSex() {
return sex;
}
public void setSex(char sex) {
this.sex = sex;
}
public int getAge() {
return age;
}
public void setAge(int age) {
if(age>120 || age<0){
this.age =3;
System.out.println("你是小朋友吗?");
}else {
this.age = age;
}
}
}
package top.oop.demo05;
public class Application {
public static void main(String[] args) {
Student student = new Student();
student.say();
student.setMoney(2);
//System.out.println(student.getMoney());
}
}
package top.oop.demo05;
// Person人 : 父类
public class Person {
//public
//protected
//default
//private
private int money = 10_0000_0000;
public void say(){
System.out.println("说了一句话");
}
public int getMoney(){
return money;
}
public void setMoney(int money){
if (money>100){
this.money = 100;
System.out.println("小兔崽子,最多给你100");
}else if(money<0){
System.out.println("输入错误");
}else if(money>0 || money<=100){
System.out.println("小兔崽子,你真乖");
}else {
this.money = 100;
}
}
}
package top.oop.demo05;
// 学生 is 人 : 派生类, 子类
// 子类继承了父类, 就会拥有父类的全部方法!
public class Student extends Person{
}
package top.oop.demo05;
// Teacher is 人
public class Teacher {
}
package top.oop.demo06;
public class Application {
public static void main(String[] args) {
Student student = new Student();
student.test("iyunsir");
student.test1();
}
}
super注意点:
1. super调用父类的构造方法,必须在构造方法的第一个
2. super必须只能出现在子类的方法或者构造方法中!
3. super和this 不能同时调用构造方法!
vs this:
代表的对象不同:
this: 本身调用者这个对象
super: 代表父类对象的应用
前提
this: 没有继承也可以使用
super: 只能在继承条件中才可以使用
构造方法
this();本类的构造
super();父类的构造
package top.oop.demo06;
//在java中, 所有的类,都默认直接或者间接的继承object
//Person人: 父类
public class Person /* extends Object */ {
public Person(){
System.out.println("Person无参执行了");
}
protected String name = "iyunsir";
//私有的东西无法被继承
public void print(){
System.out.println("Person");
}
}
package top.oop.demo06;
//学生 is 人: 派生类, 子类
// 子类继承了父类,就会拥有父类的全部方法
public class Student extends Person {
public Student(){
//隐藏代码: 调用了父类的无参
super(); //调用父类的构造器,必须要在子类构造器的第一行
System.out.println("Student无参执行了");
}
private String name = "iyunsir";
public void print(){
System.out.println("Student");
}
public void test1(){
print(); //student
this.print(); //student
super.print(); //person
}
public void test(String name){
System.out.println(this.name);
}
}
package top.oop.demo07;
//继承
public class A extends B{
//Override 重写
@Override //注解: 有功能的注释!
public void test() {
// super.test();
System.out.println("A=>test()");
}
}
package top.oop.demo07;
public class Application {
public static void main(String[] args) {
//方法的调用只和左边,定义的数据类型有关!
//静态的方法和非静态的方法区别很大
//静态方法
//非静态: 重写
A a = new A();
a.test(); //A
//父类的引用指向了子类
B b = new A(); //子类重写了父类的方法
b.test(); //B
}
}
package top.oop.demo07;
// 重写都是方法的重写,和属性无关
public class B {
public void test(){
System.out.println("B=>test()");
}
}
package top.oop.demo08;
public class Application {
public static void main(String[] args) {
//一个对象的实际类型是确定的
//new Student();
//new Person();
//可以指向的引用类型就不确定了: 父类的引用指向了子类
//Student 能调用的方法都是自己的或者继承父类的!
Student s1 = new Student();
//Person 父类型,可以指向子类,但是不能调用子类独有的方法
Person s2 = new Student();
Object s3 = new Student();
// 对象能执行那些方法,主要看对象左边的类型,和右边关系不大!
((Student) s2).eat();
s1.eat();
}
}
package top.oop.demo08;
public class Person {
public void run(){
System.out.println("run");
}
/*
多态注意事项:
1. 多态是方法的多态, 属性没有多态
2. 父类和子类,有联系 类型转换异常!ClassCastException!
3. 存在条件: 继承关系,方法需要重写,父类引用指向子类对象! Father f1 = new Son()
1. static 方法,属于类,它不属于实例
2. final 常量
3. private 方法
*/
}
package top.oop.demo08;
public class Student extends Person{
@Override //方法的重写
public void run() {
System.out.println("son");
}
public void eat(){
System.out.println("eat");
}
}
package top.oop.demo09;
public class Application {
public static void main(String[] args) {
// 类型之间的转化: 父 子
// 子类转换为父类,可能丢失自己的本来的一些方法!
//高 低
Person obj = new Student();
//Person 将这个对象转换为student类型, 我们就可以使用Student类型的方法了!
((Student) obj).go();
}
}
/*
1. 父类引用指向子类的对象
2. 把子类转换为父类,向上转型;
3. 把父类转换为子类,向下转型: 强制转换
4. 方便方法的调用,减少重复的代码!
抽象: 封装,继承,多态! 抽象类,接口
*/
/*
//Object > String
//Object > Person > Teacher
//Object > Person > Student
Object object = new Student();
//公式 System.out.println(X instanceof Y);// 能不能编译通过!
System.out.println(object instanceof Student); //true
System.out.println(object instanceof Person); //true
System.out.println(object instanceof Object); //true
System.out.println(object instanceof Teacher); //false
System.out.println(object instanceof String); //false
System.out.println("===========================");
Person person = new Student();
System.out.println(person instanceof Student); //true
System.out.println(person instanceof Person); //true
System.out.println(person instanceof Object); //true
System.out.println(person instanceof Teacher); //false
// System.out.println(person instanceof String); //false 编译报错,同级关系!
System.out.println("===========================");
Student student = new Student();
System.out.println(student instanceof Student); //true
System.out.println(student instanceof Person); //true
System.out.println(student instanceof Object); //true
// System.out.println(student instanceof Teacher); //false 编译报错,同级关系!
// System.out.println(student instanceof String); //false
}
*/
package top.oop.demo09;
public class Person {
public void run(){
System.out.println("run");
}
}
package top.oop.demo09;
public class Student extends Person{
public void go(){
}
}
package top.oop.demo09;
public class Teacher extends Person{
}
package top.oop.demo10;
public class Application {
}
package top.oop.demo10;
// final 修饰后,就不能继承!
public class Person {
//2 赋初始值
{
System.out.println("匿名代码块");
}
//1 只执行一次
static {
System.out.println("静态代码块");
}
//3
public Person(){
System.out.println("构造方法");
}
public static void main(String[] args) {
Person person = new Person();
System.out.println("===========");
Person person2 = new Person();
}
}
package top.oop.demo10;
public class Student {
private static int age; //静态的变量 多线程!
private double score; // 非静态的变量
public void run(){
}
public static void go(){
}
public static void main(String[] args){
go();
}
}
package top.oop.demo10;
// 静态导入包
import static java.lang.Math.random;
import static java.lang.Math.PI;
public class Test {
public static void main(String[] args) {
System.out.println(random());
}
}
package top.oop.demo11;
//抽象类的所有方法,继承了它的子类,都必须实现它的方法~除非~子类也是abstract,那需要子子类实现~
public class A extends Action{
@Override
public void doSomething() {
}
}
package top.oop.demo11;
// abstract 抽象类 类 extends: 单继承~ (接口可以多继承)
public abstract class Action {
//约束~ 有人帮我们实现
// abstract ,抽象方法,只有方法名字,没有方法的实现!
public abstract void doSomething();
//1. 不能new这个抽象类,只能靠子类去实现它: 约束!
//2. 抽象类可以写普通的方法
//3. 抽象方法必须在抽象类中~
//抽象的抽象: 约束~
//思考题? new,存在构造器么?
//存在的意义 抽象出来~ 提高开发效率~
}
作用:
1. 约束
2. 定义一些方法,让不同的人实现~ 10 ---> 1
3. public abstract
4. public static final
5. 接口不能被实例化~,接口中没有构造方法~
6. implements 可以实现多个接口
7. 必须要重写接口中的方法~
8. 总结博客~
package top.oop.demo12;
public interface TimeService {
void timer();
}
package top.oop.demo12;
//抽象的思维~ Java 架构师~
// interface 定义的关键字
public interface UserService {
//常量~ public static final
int AGE = 99;
//接口中的所有定义其实都是抽象的 public abstract
//void run(String name);
void add(String name);
void delete(String name);
void update(String name);
void query(String name);
}
package top.oop.demo12;
// 抽象类:extends
// 类 可以实现接口 implements 接口
// 实现了接口的类,就需要重写接空中的方法
public class UserServiceImpl implements UserService,TimeService{
@Override
public void add(String name) {
}
@Override
public void delete(String name) {
}
@Override
public void update(String name) {
}
@Override
public void query(String name) {
}
@Override
public void timer() {
}
}
package top.oop.demo13;
public class Application {
public static void main(String[] args) {
Outer outer = new Outer();
//通过这个外部类来实例化内部类~
Outer.Inner inner = outer.new Inner();
inner.in();
}
}
package top.oop.demo13;
public class Outer {
private int id=10;
public void out(){
System.out.println("这是外部类的方法");
}
public class Inner{
public void in(){
System.out.println("这是内部类的方法");
}
//获得外部类的私有属性~
public void getID(){
System.out.println(id);
}
}
}
评论一下吧
取消回复